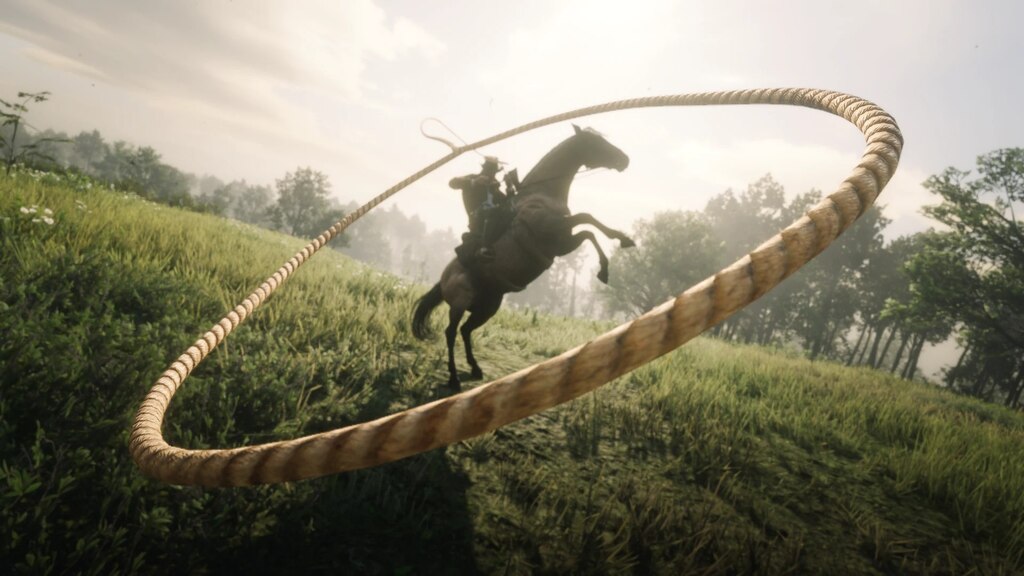
Script Overview 🪢
This script implements a realistic hogtie escape system that prevents players from breaking free when other players are nearby, enhancing roleplay immersion.
Key Features
- Automatic hogtie state detection
- Distance-based escape restriction
- Performance-optimized execution
- Configurable range settings
How It Works
The script uses two main components:
Player Range Detection
function GetPlayersInRange(range)
local playersInRange = {}
local playerPed = PlayerPedId()
local playerId = PlayerId()
local coords = GetEntityCoords(playerPed)
for _, player in ipairs(GetActivePlayers()) do
local tgt = GetPlayerPed(player)
if player ~= playerId then
local targetCoords = GetEntityCoords(tgt)
local distance = #(coords - targetCoords)
if distance <= range then
table.insert(playersInRange, GetPlayerServerId(player))
end
end
end
return playersInRange
end
Main Control Loop
Citizen.CreateThread(function()
while true do
local playerPed = PlayerPedId()
local hogtied = Citizen.InvokeNative(0x3AA24CCC0D451379, playerPed)
if hogtied then
Citizen.Wait(0)
DisableControlAction(1, 0x6E9734E8, true)
Citizen.InvokeNative(0xB8DE69D9473B7593, playerPed, 15)
local playersInRange = GetPlayersInRange(20.0)
if #playersInRange == 0 then
cantBreakFree = false
else
cantBreakFree = true
DisableControlAction(1, 0x295175BF, true)
end
else
Citizen.Wait(2000)
cantBreakFree = true
end
end
end)
Implementation Notes
Performance Optimization
- Uses dynamic wait times
- Quick updates when hogtied (0ms)
- Reduced checks when free (2000ms)
Range Configuration
- Default range: 20.0 units
- Easily adjustable for different needs
- Measured in game world units
Control Management
- Disables break-free action near players
- Uses native RedM controls
- Prevents exploit abuse
Usage Instructions
- Add the script to your server's resource folder
- Ensure proper load order in server.cfg
- Configure range value if needed
- Restart your server
Technical Details
- Native Function Calls:
0x3AA24CCC0D451379
: Hogtie state check0x6E9734E8
: Break free control0xB8DE69D9473B7593
: Ped action flags
Join Our Community!
Get help, share ideas, get free scripts, and connect with other RedM enthusiasts in our Discord server.
Join Discord